Getting Started with the TechLink API
The TechLink Services API uses a number of standard technologies, patterns, and pratices for its implementation. These are described in somewhat broad strokes below, or in detailed form in the API Documentation.
Best Practices
If you are interested in integrating your business systems with TechLink Services, here are some ways to get started.
- Familiarize yourself with TechLink's work flow and business entity relationships (work orders, job sites, user accounts, and so on).
- Identify parallels with your own business practices, design integration points into your own existing UI, and add customized elements and flow steps in order to take advantage of the TechLink API and lessen your manual workload.
- If desired, TechLink Solutions can provide custom integration for your systems.
RESTful APIs
The TechLink Services API uses RESTful architecture for its web service implementation.
You are probably familiar with the REST pattern, but if you need more information, there are a plethora of definitions, tutorials, and examples out there on the web. For a dry, academic breakdown, there's always Wikipedia, but for more intuitive, differently-scoped, sometimes brilliant, sometimes goofy information, ask Google.
The TechLink Services Work Flow
The TechLink Services work flow centers around the work order, a document detailing work to be performed for a client by an installer with their technicians. These work orders can be created via the API, once some prerequisites have been fulfilled.
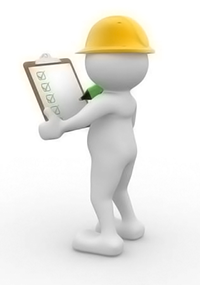
The work order will need a (job) site, a location where the work will be performed. This site can have contacts, documents, and generic attributes associated with it.
The work order can have certain user accounts associated with it in key roles (submitter, etc). The work order can also have specific tasks, documents, notes, and more associated with it. Requirements can be set on the work order for completion - job site photographs and close-out documents for the installer to submit.
So, in general, the steps are:
- sign up for TechLink Services user accounts for your key personnel
- create a job site with address and contact details
- create a work order with any associated users, requirements, and detailed instructions on the work to be performed
- relax and let TechLink Services guide the work to completion, keeping you up-to-date and informed every step of the way
Integration Examples
PHP
This page contains some examples of accessing and working with the TechLink API from various programming languages. Note that PHP and curl samples also exist on the API documentation page.
Authentication and Retrieving a Work Order in PHP
The TechLink API authentication protocol is described in detail on the API documentation page. Below is a sample API call function written in PHP. The TechLink API can be accessed using entirely native PHP functions.
A typical response to the above code follows:
JavaScript
The TechLink API can be accessed via JavaScript if an md5() encryption support function/library is included. There are plenty of JavaScript md5() implementations to choose from; use the one that best suits your needs.
Authentication and Creating a Site in JavaScript
Note that the following API access function assumes that an external md5() encryption function has been provided.
A typical response to the above code follows: